WW1 - No Man's Land Devlog 7
WW1 - No Man's Land Devlog 7
Wow, so it has been a long time since my last update on this project. Progress has been slow, but have tons to show right now, so let's get started.
Progress Overview
Firstly, here is a vid of where things are at right now:
In comparison to the progress seen last time:
Some systems seem to be missing right now, such as the card system and the dice system. The reason for this is because some major reworks had to be done to the way the battlefield is represented data-wise. In the older vid, this representation was done in a very abstract way, which made doing things like pathfinding, troop selection, etc. a bit more complex than they needed to be. The old representation also was not going to be very friendly when the time finally comes to implement the AI for the game.
So now, with that underlying system cleaned up, the final version of the game is beginning to take shape!
Custom Dev Tool
As part of the rework I made to the underlying system, I also went ahead and built myself an editor tool for quickly modifying the data for a level. While it is a bit rough, so far, it has proven to be extremely useful for debugging a TON of crap, so I think the time I invested programming it has really paid off! Certainly has saved me a ton of stress.
In the video, you can sort of see how the battlefield data is represented: it is represented with a grid, stored as a 2D array. Each color represents the grid type, of which there are several. These grid types in the end, help to dictate how troops on the battlefield will move. For example, regions that are colored in black let the troop know that this is a region in which they cannot enter, etc. From the vid, you can see this in action, where by coloring in regions black, and restarting the game, the troops walk around that region.
Still some improvements to make to the tool as well. It currently does not support undo commands, which sucks! Also, if I change the battlefield grid dimensions, I have to repaint the whole level back in, which also SUCKS! (Do not really see myself having to change grid size to often though so decided not to address this issue yet). Still, like I said, the tool has been very helpful so far, so super glad I took the time to make it, otherwise I'd have to modify a text file by hand and then reimport that into Unity and stuff, super messy and tedious!
Pathfinding
From the progress overview video, you may have noticed the pathfinding stuff that is going on with all of the troops. Behind this system is an implementation of the well known A* algorithm. This is always a fun algorithm to mess around with, since it has the effect of making your game look intelligent. At the same time, A* can be a very resource intensive algorithm in terms of it's memory usage, both when it is generating the path and when it stores the path, so optimizing it is definitely a big thing for me. At the same time, I suspect that the generated paths will, for the most part, not be extremely large since all movement will eventually have to be tied back into the dice system when I get that back up, which means a path for 1 troop can potentially only be a max amount of 12 nodes. The way the game is designed, this means that per turn, the total number of nodes stored will never exceed 12 since the number of moves is shared by the entire army! Still though, there was a simple optimization that I wanted to make, because well, it seemed simple so why not? This optimization comes directly from a youtube series by Sebastian Lague on this very algorithm. I highly recommend you watch it if you are into Unity and want to learn more about A* as well. I also recommend this video by Javidx9, especially for his explanation and walkthrough for how the algorithm works (his entire channel is pretty freaking sick actually if you are just into programming, definitely spent countless hours pouring over his C++ tutorials hehe). Anyways, here is the optimization I am referring to:
So essentially, I only need to store the nodes at which the direction changes, and the gaps in between can be filled in later; this prevents needless memory usage for nodes that can be easily computed on the run, which can prove extremely valuable I suspect when dealing with super large paths. Again though, I do not think the paths in my game will be too large, but this was a simple optimization, so I went ahead and did it for best practice anyway.
There is 1 more optimization that can be made to my A* implementation, and this is to make use of a heap data structure, which may improve the runtime of my A* implementation. This is because each time I insert unvisited nodes into my unvisited nodes collection, I need to sort this list from least global cost to most global cost, which has a linear runtime, but a heap data structure has a logarithmic runtime worst case. Still, I do not think I will actually go about doing this, just because once the dice system is in, the A* algorithm will have to search through a pretty small space pretty much all the time, so the effects of a linear runtime will probably not really be felt at all, leading me to feel like it currently is not worth it to introduce the complexity of a heap data structure in this case. We shall see though.
Troop State machine
I decided to organize my code a bit differently for each of the behaviors the troops have. Up until now, I had always just built the all the behaviors for an entity into 1 class. So for example, thinking back to my most recent project, Sengoku, for the bosses, I straight up placed all the behaviors into classes like MovementManager, etc., which resulted in these MASSIVE control flow statements. This really made debugging a pain sometimes, and tedious since I had to be scrolling A LOT just to reach specific parts of the code. So taking this into account, I decided to use a state machine: each behavior for the troops has its code stored in small containers, which also have logic for transitioning only to those states that are reachable from it, and includes the animation logic for playing the correct clip. As an example, I have a idleStanding state, and this state can only transition to shootWhileStanding, throwGrenade, deathWhileStanding, enterCrouch, etc. This will make it FAR MORE easier I believe to debug things, because now, I can easily construct debug tools for forcing a troop into a state that is having an issue, while also being able to more easily locate code that contains a bug since it is now separated very distinctly in its own file, for each behavior! Below is an image of the rough version of the state machine I designed for the troops in this game, where the arrows represent the transition logic that each state contains:
Anyways, enough technical rambling! Let's talk about some of the art I managed to make so far!
New Art
British Troops
So, I began by sketching out some potential designs for the troop sprites. Below are those early sketches. At first, I was trying to design the German troops, but somewhere along the way, I transitioned to starting things off with the British troops:
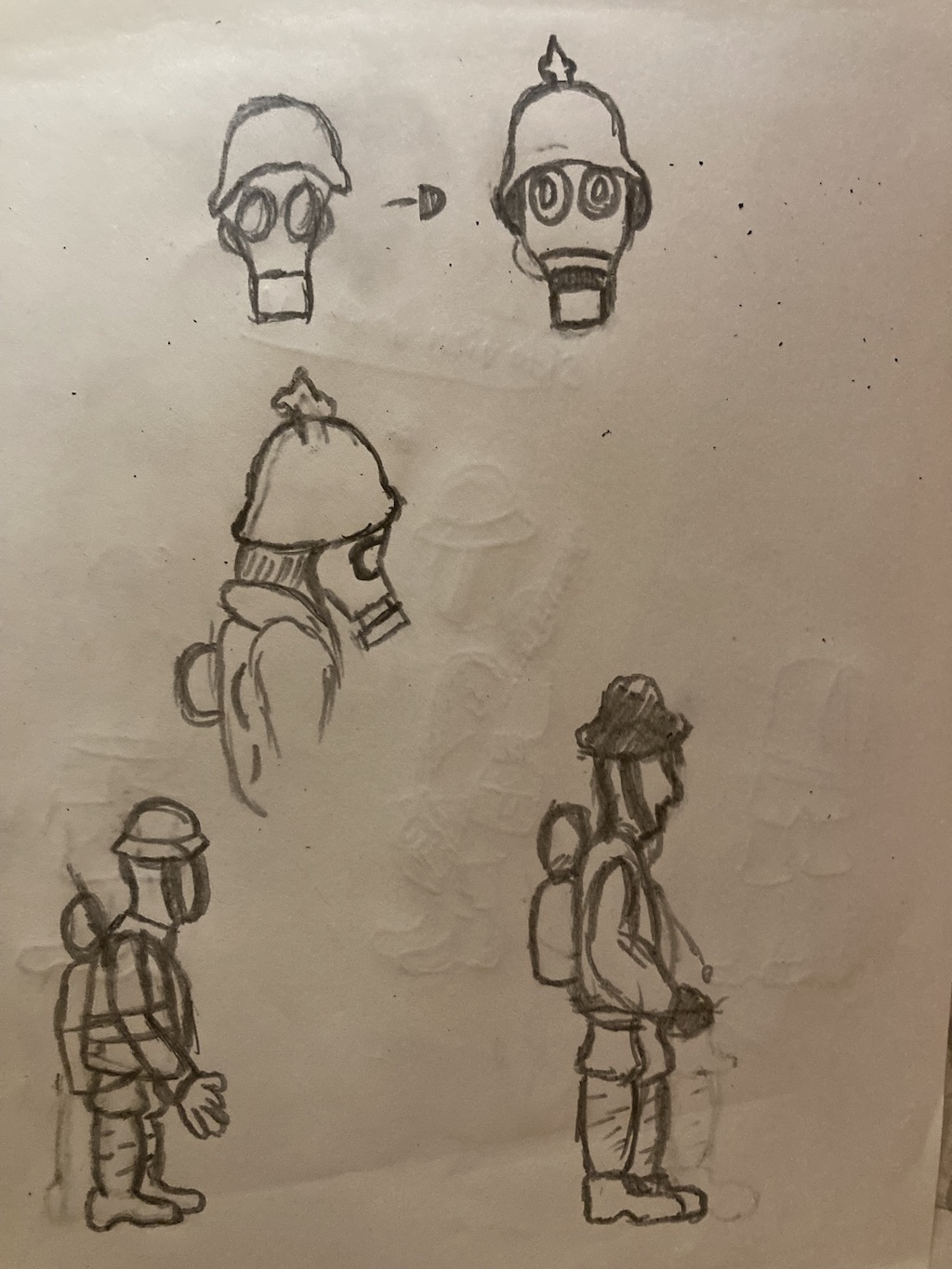
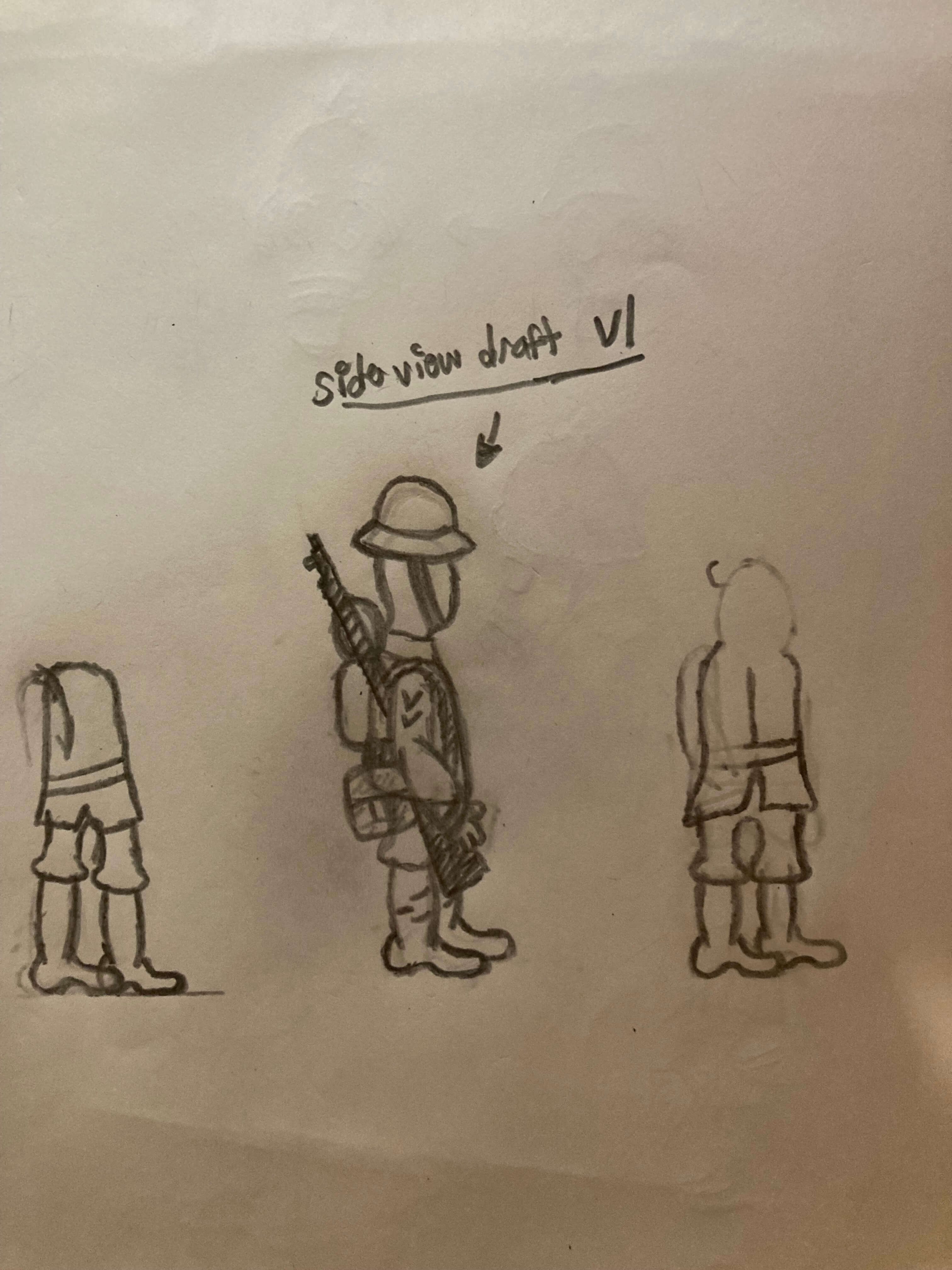
Once I reached the point seen in the 3rd image above, I began to flesh out the rest of the frames, though I still have to do the frames for looking forward and backward. Doing these frame by frame sketches is honestly where a ton of time went in, and was also extremely tedious! At the same time, it was super fun seeing the early stages of the frames that would allow this troop to come to life in the game. Below are just a couple of those sketched out frames. It was a ton of frames to be honest, I think I got at least 30-40 pages worth. I started off with stick figure poses to make sure all the movement is mapped out, and then proceeded to add the skin on top of those "bones" if you wanna think of them like that. This is why, in some of the images below, you will see stick figures drawn in or erased:
After completing the sketches for each set of animation frames, I then snapped pictures of each sheet, and imported those images into Adobe Illustrator, where I then proceeded to create the vector art versions of all the animation frames:
The reason I drew the frames first in adobe illustrator was because importing the sketches directly into the pixel art editor was extremely messy: was super hard to get a grasp of where the pixel art lines need to go since the sketches were very hard to read in some areas, and also, the sketches did not really have a uniform size for things like the helmets, height, etc, of each troop, since those were sort of roughly estimated when sketching. By using Adobe Illustrator, I was able to clean these things up, making the process of drawing them in pixel art simpler. This might not be the most efficient process - a more talented artist can probably just create all the frames directly in the pixel art editor of their choice, but I had tried doing that in a prior project (Sengoku - A time of Warriors and Demons in case you wanna check that out) and wow did that not go well haha. So, went with the slower, but effective route for myself.
The stick figure poses were also drawn up in Adobe Illustrator. The reason I did this was because prior to to even adding all the skin around these bone figures, I first imported the stick figure versions into the pixel art editor in order to ensure that the movements made sense for the type of action I was going for, otherwise, I might've ended up wasting a lot of time on an animation that looks VERY off in terms of its movement. Here are a couple of vids of what these early tests looked like:
After the Adobe Illustrator step, I then proceeded to import the frames into Piskel, where I began the work of finalizing all the details in a pixel art fashion. This was still a bit challenging, given that the conversion from vector art to pixel art was not always clean, but I think it was more easy for me personally to map out where each pixel needed to go. Below is a vid of what these adobe illustrator frames looked like prior to my final work on them:
And finally, the end results so far after all the cleanup I did:
Menus
Right now, all of my focus has been put into getting the actual core game running, so very little has been done for the menus of the game; however, early on, I did sketch out a potential idea for my main menu that I might explore further once the core game is in. I was thinking, it might be cool to have a battlefield as the background, with a Mark IV tank poking out, and then add some nice parallaxing effects, perhaps with troops running about once in a while. Not sure, nothing really decided here, but here is that sketch I made:
Tilesets
Went ahead and got some major progress done on of the tileset. At first, I was going to go with a 32x32 tile, but this was proving to be too large for the pixel perfect camera in Unity. In fact, this was 1 of the challenges I had while making this tileset: given the nature of the game, I had to ensure that the entire battlefield can fit snuggly inside of the pixel perfect camera I am using over in Unity. The camera had to be pixel perfect as well in order to insure crisp pixel art: in the recent past, on Sengoku, we had this nasty issue where the tilesets in Unity were tearing once in a while, and this was due to our camera not being the correct size for our pixel art tilesets. In short, the camera in unity has to be a specific size for tilesets to render properly, and the pixel perfect camera automatically handles the calculations for this sizing; hence, why I went with a pixel perfect camera in Unity. So, in order to ensure that the entire battlefield can fit within my pixel perfect camera, I went with a 16x16 tile for this tileset, which in turn also dictated the final dimensions of my troop frames shown above. Below, is what this tileset looks like as of now. Still planning to do another iteration on it, most notably on the area the troops actually run on top of, probably tone the texturing detail on that part way down, because it seems to be clashing with the troop frames in a way I do not really like...
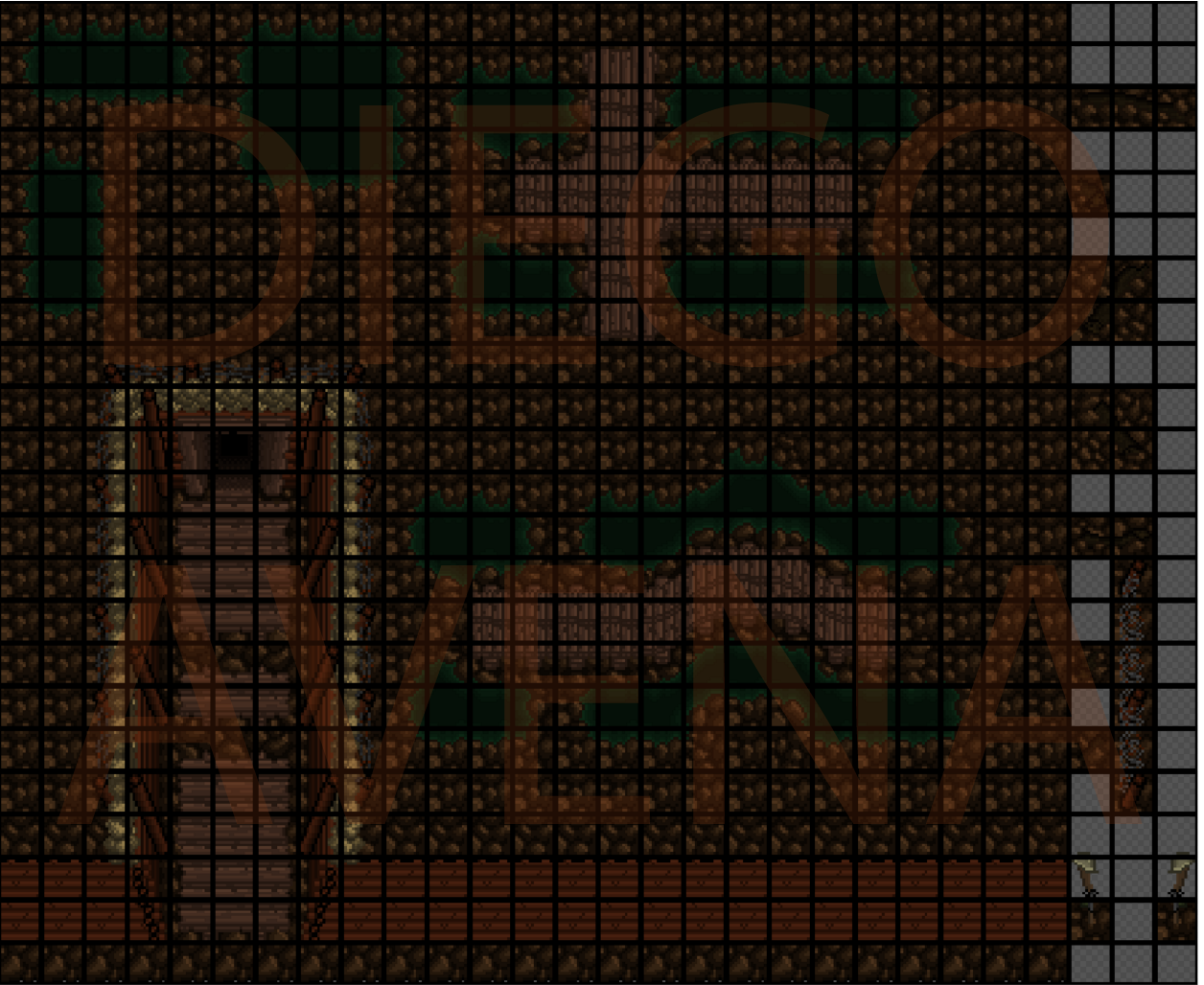
Cards and Dice
Retouched the cards from the prototype version, so now, they exist in a pixel art style. Of course, still have to get these implemented in the actual game, and I might rework a couple of them a bit just to improve their look. In addition, finally made my own version of dice, so gone are the template versions! Check it out:
Battlefield Details
Had to spice things up on the battlefield as well, so went ahead and drew a couple more sprites, some of which will not only serve for looks, but be tied to some useful game mechanics! For instance, the destroyed tree trunks, besides potentially looking cool when artillery hits them and blows them into a bunch of wood fragments, will also serve as potential areas where troops can take cover, providing players with hopefully more strategic ways to safely move their troops up toward the enemy lines!
The main control panel
I want the UI for the game to feel like it is a part of the world the game is set in, that being World War I, so I decided to make the control panel be a olive green metal panel with bolts all around it. The cards will be stored here, along with the 2 decks. Most importantly, the button for confirming all moves will be placed here, and that button is, in order to uphold my main objective with this control panel, in the form of a telegraph button! I have this whole sequence in my head where as soon as the player clicks this telegraph button, a telegraph noise plays briefly, and then you here a whistle blow as if a general is now ordering his line of troops over the trench walls, and then a type of soldiers charging plays while the troops run up the battlefield!
Closing remarks
Well, that is about all of the main things I got to show so far. Currently hard at work on getting more of the systems implemented in the game. I think the AI is going to be extremely challenging to pull off as well, but should be fun. Also gotta do some networking to allow for multiplayer mode, so that should be fun too. Looking forward to sharing more progress here! Thanks for stopping by!
WW1 - No Man's Land
A turn based game set during WW1
Status | In development |
Author | Diego A. |
Genre | Strategy, Action |
Tags | 2D, Top-Down, Turn-Based Combat, Turn-based Strategy, War, World War I |
More posts
- WW1 - No Man's Land Devlog 14Sep 03, 2023
- WW1 - No Man's Land Devlog 13Aug 07, 2023
- WW1 - No Man's Land Devlog 12Aug 04, 2023
- WW1 - No Man's Land Devlog 11Jul 09, 2023
- WW1 - No Man's Land Devlog 10Oct 15, 2022
- WW1 - No Man's Land Devlog 9Aug 10, 2022
- WW1 - No Man's Land Devlog 8Jul 14, 2022
- WW1 - No Man's Land Devlog 6Oct 30, 2021
- WW1 - No Man's Land Devlog 5Oct 23, 2021
Leave a comment
Log in with itch.io to leave a comment.